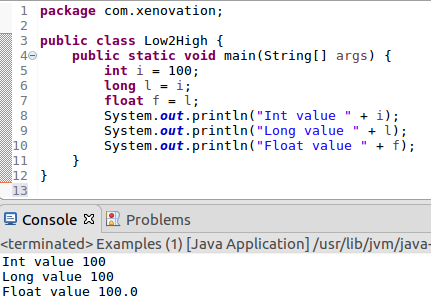
convert hex to long java
Converting Hex to Long in Java: A Beginner's Guide
Ever wondered how to transform those cool hexadecimal codes into big, usable numbers in Java? Let's dive in, and I'll walk you through the process, step by step, like a friend teaching a friend!
Understanding Hexadecimal (Hex)
Hexadecimal is a way to represent numbers using 16 symbols (0-9 and A-F). It's handy for computers, because it's a more concise way to express large binary numbers. (Imagine writing out a long phone number; shorter ways are more efficient.)
Key takeaways about Hex
- Hexadecimal uses 16 symbols, (0 to 9 and A to F) to represent any number.
- It's a shorter way to write very large binary numbers (like in memory addresses).
- A common shorthand in programming languages, often seen in colour codes (for instance, <code>#FF0000).
Understanding Long Data Type in Java
Source: xenovation.com
The long
data type in Java is used for storing big numbers. (Think very very very big numbers, much bigger than normal int
type.) You can use long
variables to save memory addresses and similar big values in programming, making everything smoother!
Source: redd.it
Long data type attributes:
long
variables can hold much larger numbers.- A long represents a large set of integers. (It’s a bigger box!)
- Long is used when you're storing really large numerical values!
Why Convert Hex to Long?
We convert hex values to long values to work with these huge values easily. (Like converting currency from a small to a huge amount of currency. ) For instance:
- Memory address management (hex to long is key to find any part in computer’s memory space).
- Storing very big amounts.
Source: medium.com
The Java Long.parseLong()
Method – The Key
The Long.parseLong()
method is your best buddy to convert a hexadecimal string to a long
value in Java. (Like having a super efficient calculator in Java itself!) Let’s look at the crucial steps.
Using Long.parseLong()
: A Simple Example
Let's try this example;
public class HexToLong {
public static void main(String[] args) {
String hexString = "FFFFFFFF"; // Example hexadecimal string
try{
long decimalValue = Long.parseLong(hexString, 16); // 16 is for hexadecimal (crucial!)
System.out.println("The long representation of " + hexString + " is: " + decimalValue);
} catch (NumberFormatException e) {
System.out.println("Invalid hexadecimal string"); // for user-friendly feedback
}
}
}
Explanation of the Example Code
Long.parseLong(hexString, 16);
: This part takes the hex string (hexString
) and the base (16
which is hexadecimal).- We've used try-catch to make sure your program does not crash for any faulty data that user might enter
Source: sourcecodester.com
Handling Errors (Crucial for Robust Code!)
What happens if the input string isn't a valid hex representation? This is where NumberFormatException
steps in! Java throws this to tell you if the value isn’t a valid number (it’s a check built in)! This is called error-handling. Here’s the revised example, with error handling:
import java.lang.NumberFormatException;
public class HexToLongWithErrors{
public static void main(String[] args) {
String hexString1 = "100A01";
try {
long value1 = Long.parseLong(hexString1, 16);
System.out.println(value1);
String hexString2 = "FGH0F"; // not valid hex
try{
long value2 = Long.parseLong(hexString2, 16);
System.out.println(value2);
}catch (NumberFormatException e) {
System.out.println("Error: Invalid hexadecimal string"); //User-friendly Error Message!
}
} catch (NumberFormatException e) {
System.out.println("Invalid Hex!");
}
}
}
The second string has error data (Invalid Input). Now our code catches this instead of crashing.
Common Mistakes and How to Avoid Them
- Wrong Base: Forgetting to use
16
as the base is very common. Make sure your code defines this! - Invalid Input: Using letters like ‘G’ in hex string could cause a number format error, make sure inputs have only characters 0-9 and A-F, to prevent program crash from mistakes
Converting Long to Hex
Now, let's see how you go from long back to hexadecimal (reverse journey!):
public class LongToHex {
public static void main(String[] args) {
long decimal = 255;
String hexString = Long.toHexString(decimal);
System.out.println(decimal + " as hex is: "+ hexString );
}
Source: ytimg.com
}
The output of this should be 255 as hex is: FF
, just to make sure you understand it’s correct and valid code.
When Should You Use It?
Remember to convert hex to long, whenever you need to treat or handle very large integer numbers in a programming context! This method helps you with this sort of challenge!